Nesting Router Outlets In Angular 6 / 7
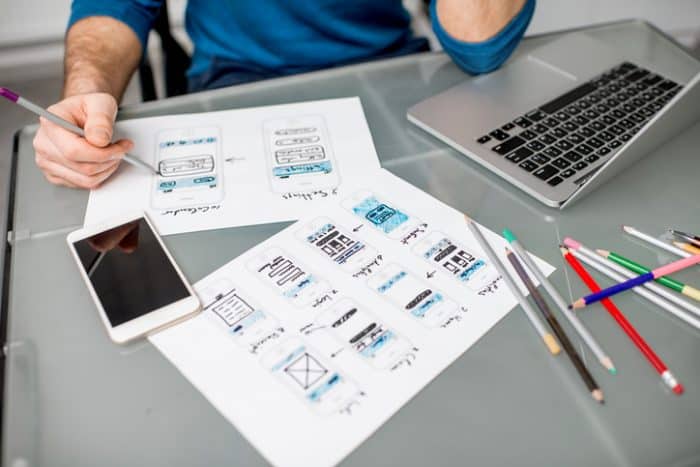
We’ve been building progressive web Applications (PWA) in Angular and Typescript for a few years now, but once in a while something new and interesting still comes along.
In the last couple of weeks I came across something pretty interesting which although is possible, is not well documented. For various reasons we are building an Angular progressive web application which has a lot of dynamically loaded components. This has led to us needing to create a nested structure out Router Outlets like this:
- Outer Component
- Router Outlet (named “outer”)
- Router Outlet (named “inner”)
- Router Outlet (named “outer”)
To make it even more fun, “outer” and “inner” both need to have parameters specified in the URLs required to access them. The overall URL needs to be copied and pasted from one browser window to another so that people can share hyperlinks. I struggled to work out two closely related things:
- How do we construct a Router Link to correctly load everything the Router Outlets?
- What does the end URL look like?
It took some patient, trial and error, but finally I worked out these critical code fragments:
Some Routing
const appRoutes: Routes = [ { path: 'outerpath/:someParameter1', component: OuterComponent, outlet: 'outer', children: [ { path: 'innerpath/:someParameter2', component: InnerComponent1, outlet: 'inner' }, { path: 'anotherinnerpath/:someParameter2', component: InnerComponent2, outlet: 'inner' } ] }];
An Example routerLink In The HTML
<a [routerLink]="['/', { outlets: { 'outer': ['outerpath', data.someParameter1, { outlets: { 'inner': ['innerpath', data.someParameter2] } }] } }]">Click Me</a>
An Example Of Using The Router To Navigate In Typescript
router.navigate( [{ outlets: { 'outer': ['outerpath', data.someParameter1, { outlets: { 'inner': ['innerpath', data.someParameter2] } } ] } }]);
An Example URL Formed Using This Approach
http://localhost:1234/someoutercomponent(outer:outerpath/123/(inner:innerpath/456))
Conclusion
Hopefully this will save you a little time guessing at RouterLink formats when you try to nest parameterised router outlets! If you are interested in co-sourcing or outsourcing your next progressive web Application, then please get in touch by filling in the form below.
Posted in: News, Progressive Web Applications