What Are Microsoft Outlook Add-Ins and How Do They Work?
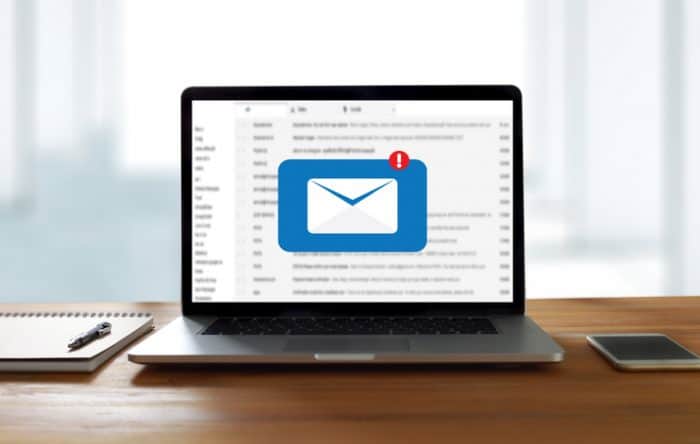
Simplifying Microsoft Office Add-In Requirement Sets
If you’ve delved into Microsoft Office Add-Ins at all, you’ve probably learned about requirement sets. Requirement sets for MS Office more or less explain the availability of API calls in the different MS Office clients. For the purposes of this article, we’re going to use the term ‘features’ to refer to the availability of API calls.
Why Are Requirement Sets Important?
Most add-ins need to run on a variety of platforms. For example, you may be building an Outlook Add-In (our favourite type of add-in) which needs to run on Outlook 2013, 2016, Microsoft 365, Office for the web, iOS and Android. Each of these platforms has a different level of support for the ‘Mailbox’ API that is commonly used in Outlook Add-Ins.
For example, the getAllInternetHeadersAsync API call was introduced in API requirement set 1.8. This means that it will only work on Office for the web, Desktop 2019+ (but not Desktop 2019 volume license) etc, so our customers using Office 2013 and 2016 can’t use this API call.
This means that your code needs to gracefully fallback if the user is using an Outlook client that does not have this API call. This is typically accomplished by hiding a piece of user interface.
How Do I Check The Requirement Set?
The syntax for checking the requirement set available on the client is a bit ugly:
Office.context.requirements.isSetSupported(“Mailbox”, “1.5”)
Imagine littering that all over your code!
To simplify things a little, we have created a ‘features’ class (or set of functions, for the functional fans) to encapsulate this logic:
export default class Features {
v1_4 = 140;
v1_5 = 150;
v1_6 = 160;
v1_7 = 170;
v1_8 = 180;
v1_9 = 190;
getMaxAPI() {
var maxApi = this.v1_4;
if (Office.context !== undefined && Office.context.requirements !== undefined) {
if (Office.context.requirements.isSetSupported(“Mailbox”, “1.5”)) {
maxApi = this.v1_5;
}
if (Office.context.requirements.isSetSupported(“Mailbox”, “1.6”)) {
maxApi = this.v1_6;
}
if (Office.context.requirements.isSetSupported(“Mailbox”, “1.7”)) {
maxApi = this.v1_7;
}
if (Office.context.requirements.isSetSupported(“Mailbox”, “1.8”)) {
maxApi = this.v1_8;
}
if (Office.context.requirements.isSetSupported(“Mailbox”, “1.9”)) {
maxApi = this.v1_9;
}
}
return maxApi;
}
isApiSupported(queryVersion) {
return queryVersion >= getMaxAPI();
}
}
Now, my syntax is much cleaner:
if (features.isApiSupported(features.v1_8))
{
…
}
What Else Can You Do With Features?
Once you’ve got your features class started, you can add other useful information to it about the office client:
isSingleSignOnSupported() {
return this.getMaxAPI() >= this.v1_6 && Office.context.mailbox.userProfile.accountType === “office365”;
}
With a bit of imagination, you can centralise all your queries for client features such as:
- platform
- is this an iPhone
- is GraphApi supported
and many other options!
For more information on the platforms and support levels of different features in an Outlook add-in, or indeed what Outlook add-ins actually are, please see the earlier posts in this series. Alternatively, please contact us to find out how we can help you with your WOPI integration.